【Python笔记(3.+)】Python记录笔记
本文全是本人自己编写
这边是目录索引,有点长,再截图中显示了就。
后面会上传Python+Selenium/appium+pytest/mysql/
等笔记文件

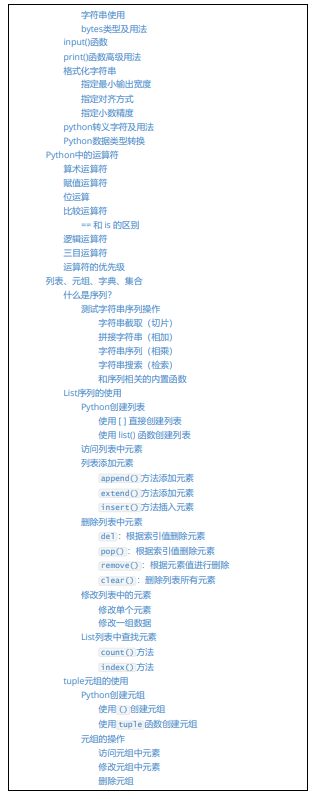

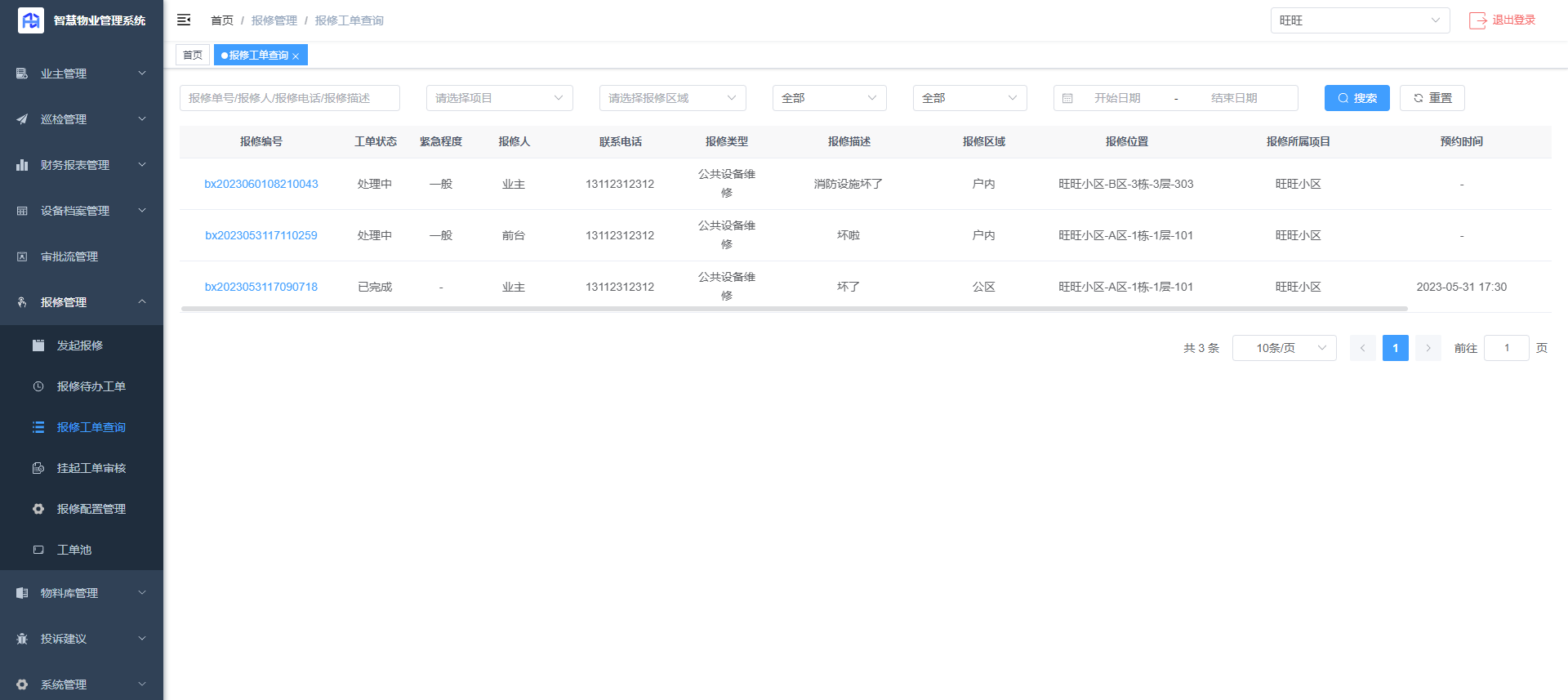
有问题的话可以回帖!
先将MySQL的一些操作贴出来,大家可以操作下!
使用的驱动可以是mysql-connector-python
里面有基本操作:(CRUD、事务处理、预编译)
Demo01
'''=================================================
home.php?mod=space&uid=155760 -> File :pythonStudy -> Db_Demo01
home.php?mod=space&uid=1557159 :PyCharm
home.php?mod=space&uid=686208 :Mr.Shi
home.php?mod=space&uid=686237 :2021/12/13 20:26
home.php?mod=space&uid=1781681 :
=================================================='''
import sys
import mysql.connector # 先安装mysql-connector 或者安装 mysql-connector-python
'''
1、导入数据库连接时需要的驱动
2、编写连接数据库所需要的配置文件信息,使用的字典序列
3、执行连接
4、测试结果
5、关闭连接释放资源
6、退出程序
'''
# 创建链接数据库 使用字典序列 编写数据库连接配置文件
config = {'host': '127.0.0.1', # 默认127.0.0.1
'user': 'root',
'password': 'root',
'port': 3306, # 默认即为3306
'database': 'examtest',
'charset': 'utf8', # 默认即为utf8,
# 将数据库的 密码编码指定为 最新的 或者说使用 -python的驱动
'auth_plugin': 'mysql_native_password',
}
cnn = None
try:
# 连接 数据库 传递 可变长参数 字典 使用 **
cnn = mysql.connector.connect(**config) # connect方法加载config的配置进行数据库的连接,完成后用一个变量进行接收
except mysql.connector.Error as e:
print('数据库链接失败!', str(e))
else:
print('success!')
finally:
cnn.close()#关闭数据库链接
sys.exit()
Demo02
'''=================================================
@Project -> File :pythonStudy -> Db_Demo01
@IDE :PyCharm
@Author :Mr.Shi
@Date :2021/12/13 20:26
@Desc :
=================================================='''
import sys
import mysql.connector # 先安装mysql-connector
# 创建链接数据库
cnn = None
try:
cnn = mysql.connector.connect(
host='127.0.0.1', # 默认127.0.0.1
user='root',
password='root',
port=3306, # 默认即为3306
database='examtest',
charset='utf8', # 默认即为utf8,
auth_plugin='mysql_native_password'
) # connect方法加载config的配置进行数据库的连接,完成后用一个变量进行接收
except mysql.connector.Error as e:
print('数据库链接失败!', str(e))
else:
print('success!')
finally:
cnn.close()
sys.exit()
查询SQL
无参查询
'''=================================================
@Project -> File :pythonStudy -> Db_Demo01
@IDE :PyCharm
@Author :Mr.Shi
@Date :2021/12/13 20:26
@Desc :
=================================================='''
import sys
import mysql.connector # 先安装mysql-connector
# 创建链接数据库
cnn = None
cursor = None
try:
cnn = mysql.connector.connect(
host='127.0.0.1', # 默认127.0.0.1
user='root',
password='root',
port=3306, # 默认即为3306
database='examtest',
charset='utf8', # 默认即为utf8,
auth_plugin='mysql_native_password'
) # connect方法加载config的配置进行数据库的连接,完成后用一个变量进行接收
except mysql.connector.Error as e:
print('数据库链接失败!', str(e))
else:
# 编写sql 语句
sql = "select * from students"
# 创建 光标 来执行 sql
cursor = cnn.cursor()
# 执行sql 来查询结果
cursor.execute(sql)
# 获取结果中全部的值 赋值 给 value 以列表的形式展示
value = cursor.fetchall()
print(value)
finally:
# 释放资源
cursor.close()
cnn.close()
# 退出程序
sys.exit()
创建表操作
在 python 中 可以编写创建 表 的sql 语句来进行 创建表操作。
以及增删改查
工具类
'''=================================================
@Project -> File :pythonStudy -> DbConnect
@IDE :PyCharm
@Author :Mr.Shi
@Date :2021/12/15 19:03
@Desc :简单定义一个 连接数据库的工具类
=================================================='''
import mysql
def con():
try:
cnn = mysql.connector.connect(
host='127.0.0.1', # 默认127.0.0.1
user='root',
password='root',
port=3306, # 默认即为3306
database='examtest',
charset='utf8', # 默认即为utf8,
auth_plugin='mysql_native_password'
) # connect方法加载config的配置进行数据库的连接,完成后用一个变量进行接收
except mysql.connector.Error as e:
print('数据库链接失败!', str(e))
else:
return cnn
测试
'''=================================================
@Project -> File :pythonStudy -> Db_Demo01
@IDE :PyCharm
@Author :Mr.Shi
@Date :2021/12/13 20:26
@Desc :
=================================================='''
import sys
# 如果是 调用的 工具类的话,在当前类中 也要使用 import 将连接数据库所需要的包 导入进来
import mysql.connector
from util import DbConnect
'''
# 使用 python 中的函数 创建一个 创建sql 表的 函数
'''
def create_table():
# 创建链接对象 通过 调用 封装好的 类 来进行 连接数据库
cnn = DbConnect.con()
cursor = None
# 创建 光标 来执行 sql
try:
# 编写 一条 创建表的 sql 语句
sql = 'create table `python_test_sql`(' \
'id int not null comment \'id编号\',' \
'name varchar(255) null comment \'姓名\'' \
');'
cursor = cnn.cursor()
# 执行sql 来查询结果
cursor.execute(sql)
except mysql.connector.errors as e:
print("创建表失败", str(e))
# 释放资源
cursor.close()
cnn.close()
# 退出程序
sys.exit()
'''
# 创建一个 向表中 添加数据的 方法
1、使用 字符串传递参数的方式进行 参数的 传递
'''
def insert_content_str(id, name):
cnn = DbConnect.con()
# 通过连接数据库的方法 cnn 创建 游标
cursor = cnn.cursor()
# 编写 插入数据的 sql 的语句
sql = "insert into python_test_sql(`id`,`name`) values (" + str(id) + ",'" + name + "')"
print(sql)
cursor.execute(sql)
# 如果 数据库 mysql 的引擎使用的是 innodb 需要提交事务
# 1.
# cnn.commit()
# 2.
cursor.execute('commit')
sys.exit()
'''
2、在向数据库中表 添加数据时 可以使用 占位符来进行传参 使用元组 来 将占位符 替换掉即可
'''
def insert_content_tuple(data):
cnn = DbConnect.con()
cursor = cnn.cursor()
sql = "insert into python_test_sql(`id`,`name`) values (%s,%s)"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql, data)
cnn.commit()
sys.exit()
'''
3、在 python中支持 多行插入 使用的 是 列表 序列
'''
def insert_content_list(data):
cnn = DbConnect.con()
cursor = cnn.cursor()
sql = "insert into python_test_sql(`id`,`name`) values (%s,%s)"
# 执行 插入 多天记录 到 数据库中
cursor.executemany(sql, data)
cnn.commit()
sys.exit()
'''
查询 id 为 1 的 所有的记录数
'''
def selectById(id):
cnn = DbConnect.con()
cursor = cnn.cursor()
sql = "select * from python_test_sql where id =%s"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql, id)
# 获得 sql 执行完毕的结果
value = cursor.fetchall()
print(value)
sys.exit()
'''
删除 某一条记录
'''
def delectById(id):
cnn = DbConnect.con()
cursor = cnn.cursor()
sql = "delete from python_test_sql where id =%s"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql, id)
cnn.commit()
sys.exit()
'''
修改 某一条记录
'''
def updateById(data):
cnn = DbConnect.con()
cursor = cnn.cursor()
sql = "update python_test_sql set id = %s where id =%s"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql, data)
cnn.commit()
sys.exit()
if __name__ == '__main__':
# create_table()
# insert_content_str(1, "hanamaki")
# 定义一个元组
# tuple_data = ("2", "andy",)
# insert_content_tuple(tuple_data)
# 定义一个 列表序列
# list_data = [
# ("3", "jojo"),
# ("4", "rose")
# ]
# insert_content_list(list_data)
# selectById((1,))
# delectById((1,))
updateById((1, 2))
事务的操作
'''
事务的操作
'''
def transActions(transferPersonId, payeeId, money):
print(transferPersonId,payeeId,money)
cnn = DbConnect.con()
cursor = cnn.cursor()
# 建表操作
create_sql = 'create table `transActions_sql`(' \
'transferPerson int ,' \
'money int' \
')ENGINE=Innodb DEFAULT CHARSET = utf8'
# MyISAM 和 innodb 不一样 增 删 改 不需要 操作 事务
cursor.execute(create_sql)
# 创建表完成之后我们可以开始 向表中先添加两列值
sql = "insert into transActions_sql(`transferPerson`,`money`) values (%s,%s)"
# 执行 插入 多天记录 到 数据库中
data = [(1, 5000), (2, 4000)]
cursor.executemany(sql, data)
cnn.commit()
print("-------------------")
# 当表中的数据创建完成之后 开始 事务的操作
try:
print("+++++++++++++++++++++++")
sql1 = "update transActions_sql set money = money - %s where transferPerson =%s"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql1, (money, transferPersonId,))
cnn.commit()
sql2 = "update transActions_sql set money = money + %s where transferPerson =%s"
# 通过 执行sql 时 可以将 数据传递给 sql 中的占位符
cursor.execute(sql2, (money, payeeId,))
cnn.commit()
print("成功")
except :
print("失败")
cnn.rollback()
finally:
print(")))))))))))))))))))))))))")
sys.exit()
调用
transActions(1, 2, 500)
将PDF文件放在链接地址中了,下载即可